2D Engine in C++
The goal
Creating a 2D engine and a small game to prove that it works. By using Open GL as a graphics renderer and some Game Programming Patterns (Robert Nystrom), Pengo an 80's arcade came to life.
The process
As mentioned above, Game Programming Patterns, a book written by Robert Nystrom, was often the red wire through this project. The following patterns were implemented in the engine:
- Observer: a seperate object that gets triggered once an event occurs, such as a kill. Once triggered, it will execute a certain action.
- State: input will be handled seperately and depending on the current state the player is in, it will return a new or the same state. If it's the same state, then nothing will happen. If it's another state, an enter function will be called, changing for instance the graphics of the character.
- Component: probably one of the most important patterns is this one. The component system allows to completely seperate functionality of different domains from each other, without them intervening. However one entity can still span over these multiple domains.
- Update Method: super simple, but super necessary. This pattern is about Game Objects having their own Update function which will be called during the gameloop. In our case, this update function will call the components' update function (components are attached to a Game Object). Objects get a 'Destroyed' tag, so that an update won't be executed during that frame.
- Game Loop: probably found in every game. A game loop processes the input of the player, it updates all Objects in the scene and it renders what's needed to come on the screen. This gameloop, makes sure the game runs at a consistent framerate or it makes sure that on an slower computer, the same amount of stuff happens in the same amount of real time. What I added as well is when there is time left (that means a loop went faster than the max frame-time), rather than sleeping, objects that are destroyed will be deleted in memory.
Other things added is the A* algorithm, but simplified. This algorithm is used for the enemy to travel towards its prey. Also the maze is procedurally generated by using the Prim's algorithm.
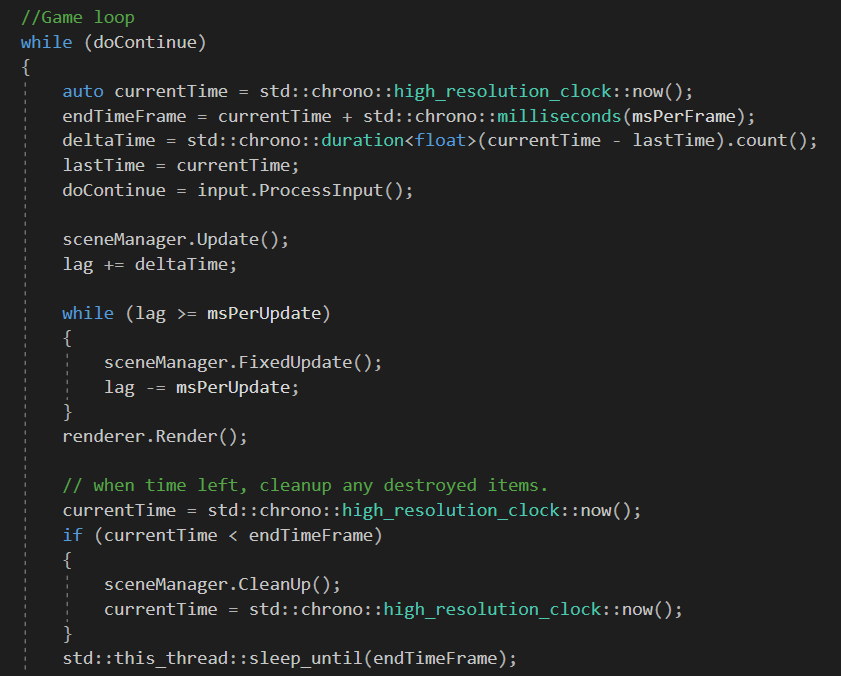
The Game Loop.
Conclusion and take aways
This was probably one of the better projects in order to understand engine design and get a better grasp on C++. By making the game I got to know two new algorithms which I never implemented before.